Update! FormDen can now automatically validate your form fields fields for you. Learn how!
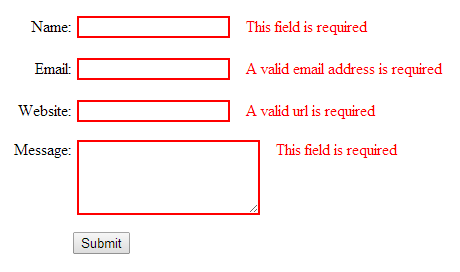
People make mistakes when they fill out forms. By validating form responses before accepting them, we can alert users to their errors before they submit the form. In this way, client side form validation can vastly improve the user experience. This article will explain some basics about form validation and then illustrate how to validate form fields using jQuery.
Use JavaScript Validation to improve UX
There are two types of validation: client side validation and server side validation. In client side validation the data is validated in the browser using JavaScript before submitting the data to the server. For example, suppose your user submits the form without entering a mandatory field. If you validate the form using jQuery, you can notice this and alert the user to their mistake instead of submitting the form. JavaScript form validation is a great way to help your users avoid mistakes when filling out a form
Don't use JavaScript Validation for Security!
Alternatively, if you want to protect your server from malicious users, then you should use server side validation because JavaScript can be easily bypassed. For example, suppose you wanted to validate a credit card. All credit cards are 16 digits and the 16th digit can be calculated based on the first 15 numbers. This means you can use JavaScript to check for, and alert the user to, credit card typos. However, to see whether a credit card has sufficient funds requires communicating with the credit card company and that can only be done using a server side programming language. Similarly, form data is typically saved in a database on the server. Because your database contains sensitive information, hackers may attempt to use your form to submit malicious commands to your backend database. If you are using a MySQL database, this is called MySQL Injection. To avoid this problem, you must validate form submissions using your server. You cannot rely on JavaScript to avoid this security problem.
Demo
Try out our demo below to test our validation!
You can also download this demo and check out the source as we go through this tutorial.
Our form contains two types of validation.
Real-Time Validation
Validate each field as it is being entered. Valid inputs will turn green while invalid inputs will turn red.

Submission Validation
When the submit button is pushed, jQuery will check whether all fields are valid. If any fields are not valid, the form will not be submitted and the user will be informed with error messages for the fields that are causing problems.
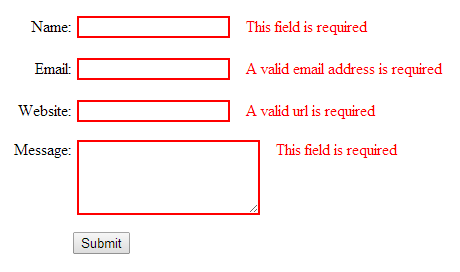
In this tutorial, we will check that:
- The name field has been filled out.
- The email address field looks like an email address.
- The webpage field looks like a url.
- The message field has been filled out.
To get started, we'll create an HTML page that include a form with the id "contact". Each input will be contained in a div. It should contain an label, an input (or textarea), and a span that will contain the error message. The form in HTML will look like this:
<form id="contact" method="post" action="">
<!-- Name -->
<div>
<label for="contact_name">Name:</label>
<input type="text" id="contact_name" name="name"></input>
<span class="error">This field is required</span>
</div>
<!-- Email -->
<div>
<label for="contact_email">Email:</label>
<input type="email" id="contact_email" name="email"></input>
<span class="error">A valid email address is required</span>
</div>
<!--Website -->
<div>
<label for="contact_website">Website:</label>
<input type="url" id="contact_website" name="website"></input>
<span class="error">A valid url is required</span>
</div>
<!-- Message -->
<div>
<label for="contact_message">Message:</label>
<textarea id="contact_message" name="message"></textarea>
<span class="error">This field is required</span>
</div>
<!-- Submit Button -->
<div id="contact_submit">
<button type="submit">Submit</button>
</div>
</form>
Note that the <label> tag improves usability. Clicking on the label will move the mouse cursor to the input with the id specified in the for attribute. The name of each input (or textarea) will be passed to the server to identify the contents of the form. Finally, notice that instead of using "text" as the input type for the email and url fields, we use "email" and "url". This will buy us free validation from browsers that support HTML5 even if JavaScript is turned off. However, if JavaScript is turned on, our validation will solve any problems before the browser sees them.
We apply a little CSS to align the labels and space out the fields:
#contact label{
display: inline-block;
width: 100px;
text-align: right;
}
#contact_submit{
padding-left: 100px;
}
#contact div{
margin-top: 1em;
}
textarea{
vertical-align: top;
height: 5em;
}
The error messages should only be shown when a field has been entered incorrectly. Therefore, we'll hide them by default. After the form is submitted, we can reveal the error message by changing the class name with JavaScript.
.error{
display: none;
margin-left: 10px;
}
.error_show{
color: red;
margin-left: 10px;
}
These error message will only be displayed after the form is submitted. We also want to implement real-time validation where the color of the input will change based on the validity of the data that has been entered. If the data is valid the input box should be green. If the data is invalid, the input box should be red. Therefore, we'll create CSS classes to represent these possible outcomes.
input.invalid, textarea.invalid{
border: 2px solid red;
}
input.valid, textarea.valid{
border: 2px solid green;
}
Set-up jQuery
Now that we've created a working form, we need to add some jQuery and JavaScript to validate fields and change the CSS classes that were created earlier. If you haven't already, make sure that you've included the jQuery library on your page:
<script type="text/javascript" src="jquery.min.js"></script>
Wrap all of the subsequent jQuery code with:
$(document).ready(function() {
//jQuery code goes here
});
so that our scripts are loaded after the page has finished loading.
Use jQuery to Validate in Real-time
We can detect that a user has started entering their name into the name input with:
$('#contact_name').on('input', function() {
});
Since the name field is required, we simply need to check whether a value for the input exists. If it exists, we'll apply the valid class and remove any invalid class. This will place a green border around the input box. The full code looks like this:
// Name can't be blank
$('#contact_name').on('input', function() {
var input=$(this);
var is_name=input.val();
if(is_name){input.removeClass("invalid").addClass("valid");}
else{input.removeClass("valid").addClass("invalid");}
});
The same approach can be used to validate the email address. The only difference is that now we want to check whether the email address looks like an email address. We know that email addresses should include an @ symbol and end with a feasible domain name. To test this, we can use regular expressions. Regular expressions are a programming language for parsing text. Fully understanding them is outside of the scope of this tutorial. However, the basic idea is to specify a pattern and test that pattern against a string of characters. If the test passes, the string is an email address. If it fails, the string is not an email address. The class is adjusted according. All of this is achieved with the following code:
// Email must be an email
$('#contact_email').on('input', function() {
var input=$(this);
var re = /^[a-zA-Z0-9.!#$%&'*+/=?^_`{|}~-]+@[a-zA-Z0-9-]+(?:\.[a-zA-Z0-9-]+)*$/;
var is_email=re.test(input.val());
if(is_email){input.removeClass("invalid").addClass("valid");}
else{input.removeClass("valid").addClass("invalid");}
});
A symmetrical approach is used to validate a url. The main difference is that the pattern to identify a url is different from the pattern to identify a website. Also, a valid url must start with http:// but many people omit this and just use www. To help them out, the code will automatically replace www. with http://www. The validation code is as follows:
// Website must be a website
$('#contact_website').on('input', function() {
var input=$(this);
if (input.val().substring(0,4)=='www.'){input.val('http://www.'+input.val().substring(4));}
var re = /(http|ftp|https):\/\/[\w-]+(\.[\w-]+)+([\w.,@?^=%&:\/~+#-]*[\w@?^=%&\/~+#-])?/;
var is_url=re.test(input.val());
if(is_url){input.removeClass("invalid").addClass("valid");}
else{input.removeClass("valid").addClass("invalid");}
});
Finally, the message validation only requires that something be entered. The code is nearly identical to the name validation:
// Message can't be blank
$('#contact_message').keyup(function(event) {
var input=$(this);
var message=$(this).val();
console.log(message);
if(message){input.removeClass("invalid").addClass("valid");}
else{input.removeClass("valid").addClass("invalid");}
});
Use jQuery to Validate after Submission
Hopefully, your users should be able to use the real-time validation to see whether they have made any errors. However, it's possible they don't notice the red box or understand why their input is not validating. Therefore, we'll double check that all the fields are valid when the submit button is pushed. If a field is not valid, we'll display the error message.
We can capture the submit button being clicked with:
$("#contact_submit button").click(function(event){
});
Then, we get an array of all of the data that has been submitted. We'll loop through every input in the form. If it does not contain the CSS class "valid", we know that the real-time validation script has found an error in an input. Therefore, we'll change the class from "error" (which is hidden), to "error_show" to display the error message explicitly describing what went wrong. In jQuery, this looks like:
// After Form Submitted Validation
$("#contact_submit button").click(function(event){
var form_data=$("#contact").serializeArray();
var error_free=true;
for (var input in form_data){
var element=$("#contact_"+form_data[input]['name']);
var valid=element.hasClass("valid");
var error_element=$("span", element.parent());
if (!valid){error_element.removeClass("error").addClass("error_show"); error_free=false;}
else{error_element.removeClass("error_show").addClass("error");}
}
if (!error_free){
event.preventDefault();
}
else{
alert('No errors: Form will be submitted');
}
});
If there are any problems, we'll prevent the form from submitting. Otherwise, it will submit itself. In this example, we're not dealing with any server side processing. So, we'll simply issue a JavaScript alert to say that everything worked.
You may notice that it would be very easy for someone to get around our validation. They could just change the class on one of the inputs from invalid to valid. This illustrates that JavaScript validation is inherently insecure. Don't rely on it for security. However, it can vastly improve the user experience.